PDF Toolbox SDK
Dokumentation & Codebeispiele
Linux
MacOS
Windows Client
API
GUI
.NET Core
NuGet
C/C++
Java
Entwicklerhandbücher & Referenzen
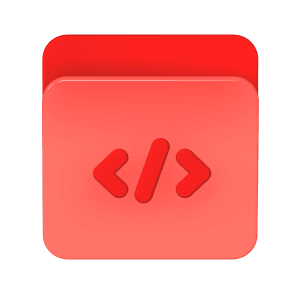
PDF Toolkit Java-Referenz
Sehen Sie sich die Referenzdokumentation für Java an, um mit der Entwicklung des PDF Toolkit SDK zu beginnen.
Völlig risikofrei
Schalten Sie Ihre PDF-Superkräfte frei
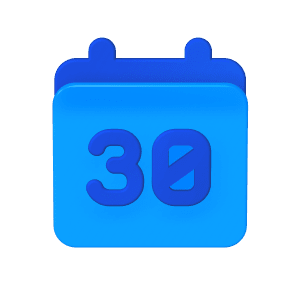

