PDF to PDF/A Converter
Documentation & code examples
Linux
MacOS
Windows Client
Windows Server
API
Shell tool (command line)
Watched folder (Windows only)
Java
C#
.NET Core
C/C++
Developer guides & references
Component CodeSamples has not been created yet …
Testen Sie es völlig risikofrei
Es ist schnell und einfach, damit anzufangen
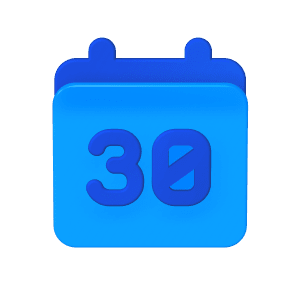

