PDF Security
Documentation & code samples
Linux
MacOS
Windows Client
Windows Server
API
Shell tool (command line)
Watched folder (Windows only)
C/C++
Java
C#
.NET Core
Developer guides & references
Component CodeSamples has not been created yet …
Testen Sie es völlig risikofrei
Es ist schnell und einfach, damit anzufangen
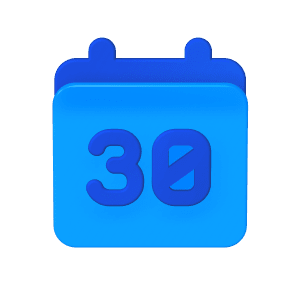

