PDF Validator
Exemples de documentation et de code
Linux
MacOS
Client Windows
Serveur Windows
API
Outil Shell (ligne de commande)
Dossier surveillé (Windows uniquement)
Java
C#
.NET Core
C/C++
Guides et références des développeurs
Component CodeSamples has not been created yet …
Essayez-le complètement sans risque
Il est rapide et facile de commencer
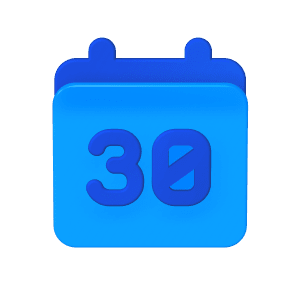

